1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| package org.Solution.Algorithm;
import java.util.concurrent.atomic.AtomicInteger;
public class Third { private static Integer i = 0; private static AtomicInteger atomicI = new AtomicInteger(0);
public static void main(String[] args) throws InterruptedException { Thread unsafeThread = new Thread(() -> { for (int j = 0; j < 100; j++) { synchronized (i){ i++; } } }); Thread unsafeThread1 = new Thread(() -> { for (int j = 0; j < 100; j++) { synchronized (i){ i--; } } });
unsafeThread.start(); unsafeThread1.start();
unsafeThread.join(); unsafeThread1.join();
Thread.sleep(1000); System.out.println("Unsafe i: " + i); } }
|
当循环次数在127以内时,每次执行完毕i一定是0,这是由synchronized保证的。
但是循环次数大于127呢?
i是一个Integer对象。在Java中,自动装箱的Integer对象在作为同步锁时,由于其值可能在内存中不唯一(因为Integer缓存了-128到127之间的值,超出这个范围的值才会每次创建新的对象),
可能导致意料之外的同步行为。
附上两个运行结果:

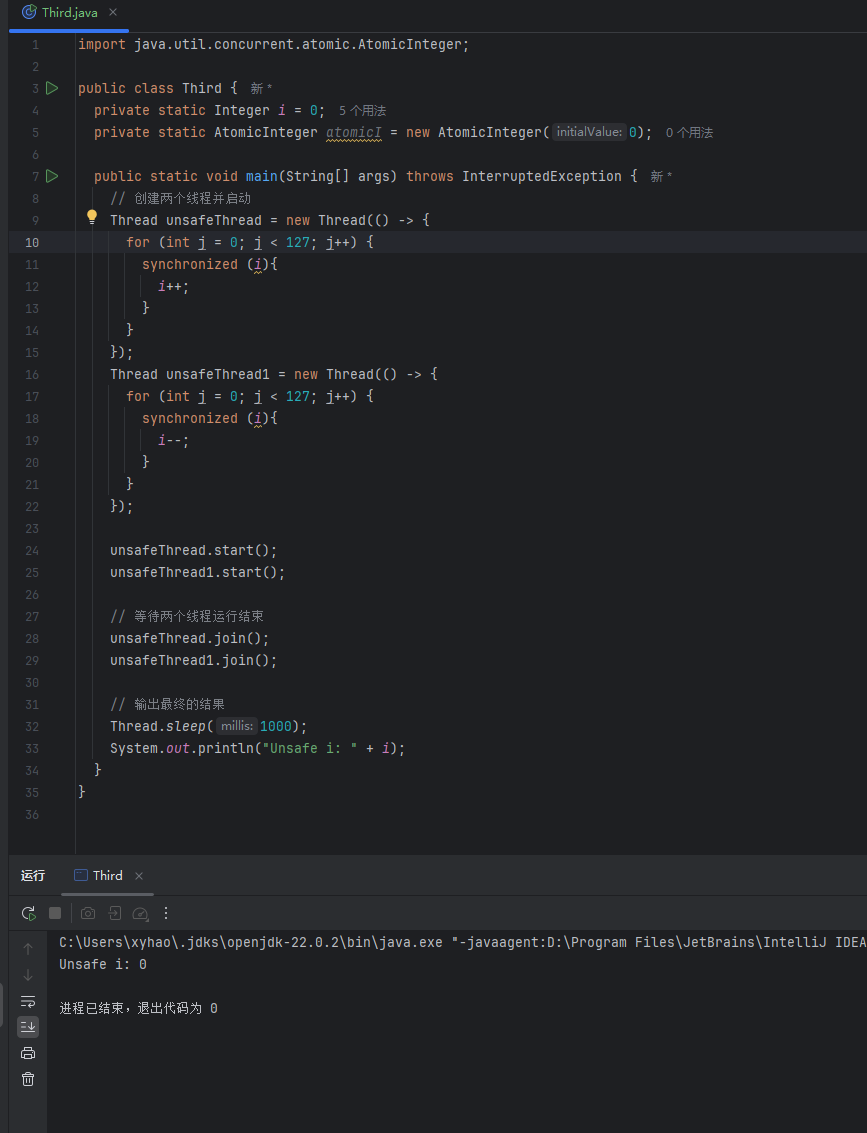